python进阶中需要深入理解python中一切皆对象,只有理解python中一切皆对象后,才能在python编程中越走越远。
1.一切皆对象
在python
中一切皆对象,包括函数
、类
、方法
都是对象,但是函数和类比较高级,属于python中的一等公民,具有以下属性
一等公民具有属性:
- 可以赋值给一个变量
- 可以添加到集合对象中
- 可以作为参数传递
- 可以当做函数的返回值
函数和类赋值给一个变量
def hello():
print("hello world")
class Person:
def __init__(self):
print("person")
h = hello
P1 = Person
h()
p1()
函数和类可以添加到集合对象中
def hello():
print("hello world")
class Person:
def __init__(self):
print("person")
li = []
li.append(hello)
li.append(Person)
#执行
for item in li:
item()
函数和类可以作为参数传递
def hello(fn):
fn()
def test():
print("我是一个测试函数")
class Person:
def __init__(self):
print("person")
#函数作为值传递
hello(test)
#类作为值传递
hello(Person)
函数和类可以作为返回值
def test():
print("test")
class Person:
def __init__(self):
print("person")
def hello():
return test
def testClass():
return Person
#函数作为返回值
hello()
#类作为返回值
testClass()
2.type、class、object三者关系
什么是对象?对象就是一个实体,如一张桌子,一把椅子等等,只要是一个具体的实物他就是一个对象。在理解对象后,接下来在理解type
、class
、object
三者之间的关系。
tpye
type有两种用法:
type()和isinstance()的区别:
- type() 不会考虑继承关系
- isinstance() 会考虑继承关系
type和class关系
a = 1
type(a)
>>> <class 'int'>
type(int)
>>> <class 'type'>
st = 'abc'
type(st)
>>> <class 'str'>
type(str)
>>> <class 'type'>
通过上面的例子,可以得出 type
-> int
-> 1
也就是 type
-> class
-> obj
(这个obj就是我们经常说的实例也就是一个具体的对象),我们可以验证下自己定义的类是不是满足
class Student:
pass
stu = Student()
type(stu)
>>> <class '__main__.Student'>
type(Student)
>>> <class 'type'>
通过我们自定义student
类发现也满足type
-> class
-> obj
的关系。
class和object的关系
#python 2版本定义类
class Student(object):
pass
#python 3版本定义类
class Student:
pass
通过对比python2版本和python3版本中定义类,发现在python 3版本中如果不声明继承基类(也就是父类),则默认继承objec。下面我们来验证一下
print(int.__bases__)
>>> <class 'object'>
print(str.__bases__)
>>> <class 'object'>
#自定义类
class Person:
pass
class Student(Person):
pass
print(Person.__bases__)
>>> <class 'object'>
print(Student.__bases__)
>>> <class '__main__.Person'>
type和object的关系
type(type)
>>> <class 'type'>
type.__bases__
>>> (<class 'object'>,)
type(object)
>>> <class 'type'>
object.__bases__
>>> ()
通过上面例子,我们可以发现 type的类就是type
,继承object
。object的类也是type
,无继承。object是python中最顶层的类,所有的类都继承于object
。
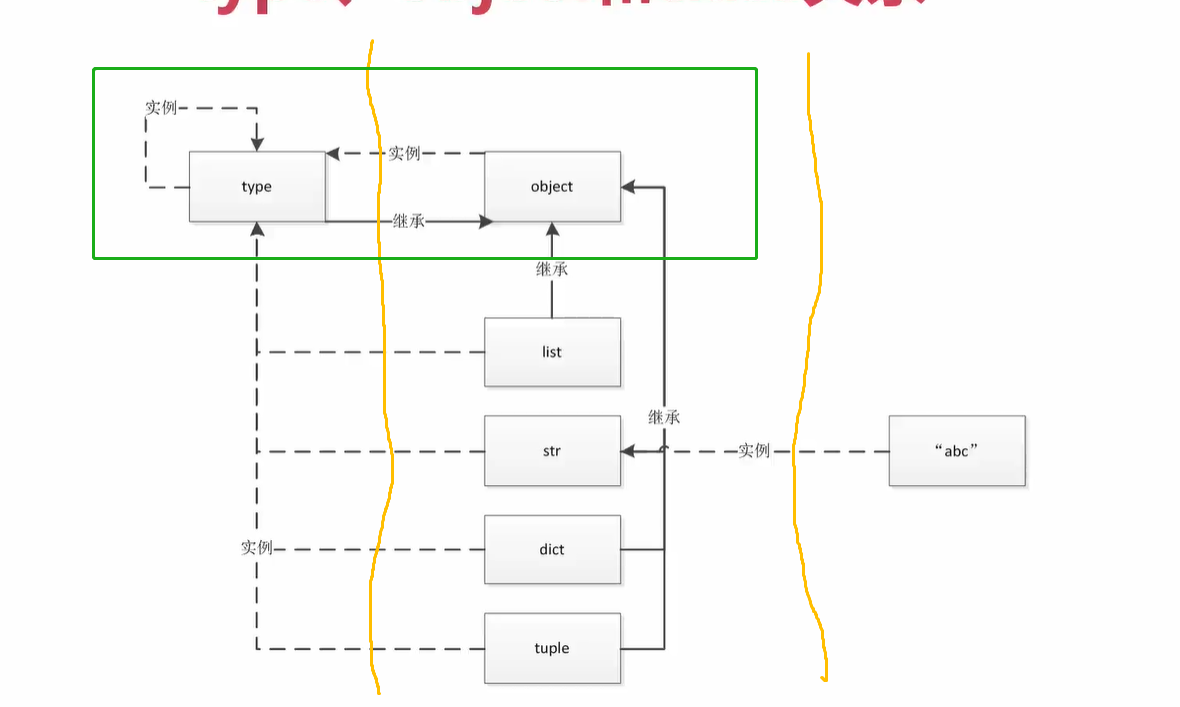
上面这张图我把它分为2部分,第一部分是用绿色框起来的,第二部分是用黄色线分开的。为了更好的理解这张图,我们先来看黄色线分开的这部分。
第二部分关系:abc 是 str的实例,str继承object, str是type实例,这部分理解起来比较容易,开头说过type的作用一种是生成类型,一种是返回对象的类型,在后面会详细介绍如何用type生成自定义的类
第一部分关系,type可以实例type, object 是type的实例,也就是type是python中用于生成实例的,type继承objec,object继承(),说明object是python最顶层的类。到这里小伙伴们应该理解了python一切皆对象
这句话的含义了
3.python的内置类型
python中对象有三个特性,身份
(在内存中的地址)、类型
(类型)、值
a = 1
id(a)
>>> 1533568176
type(a)
>>> <class 'int'>
#值
1
python中的内置类型:
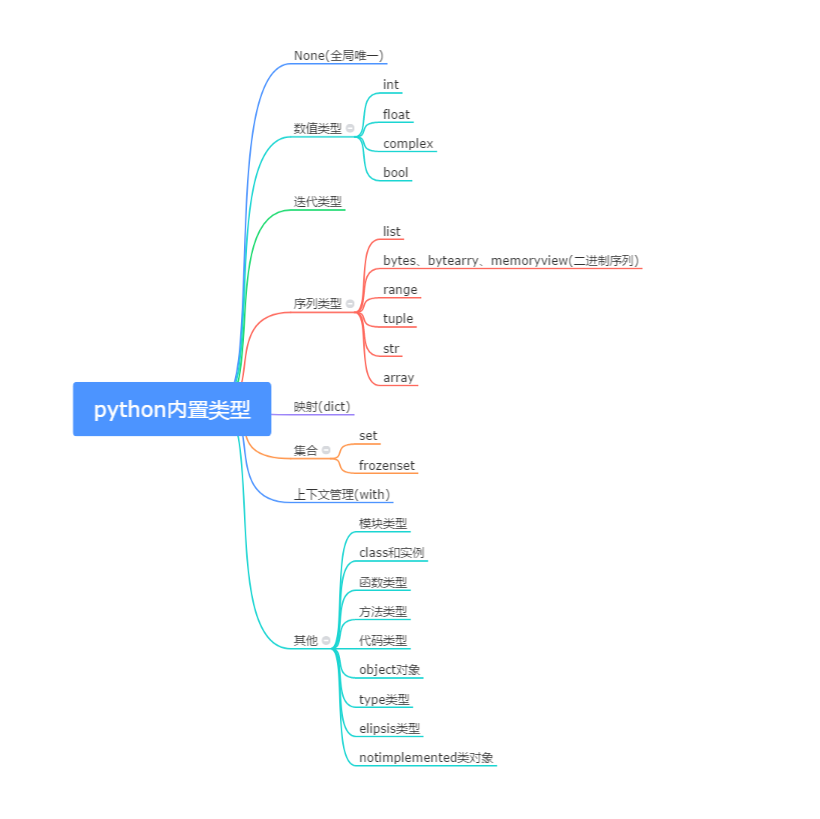